티스토리 뷰
HTTP 메세지 규약
1. 시작줄 (start line)
Response에선 상태줄(status line)
2. 헤더
3. 본문
-
- Request 메시지
- 시작줄: API 요청 내용
- GET **naver.com** HTTP/1.1
- 헤더(메타 정보, 추가적인 정보)
- "Content type"
- 없음
- HTML <form> 태그로 요청 시 Content type: application/x-www-form-urlencoded
- AJAX 요청 Content type: application/json
- "Content type"
- 본문
- GET 요청 시: (보통) 없음
- POST 요청 시: (보통) 사용자가 입력한 폼 데이터 name=홍길동&age=20
- Response 메시지
- 상태줄: API 요청 결과 (상태 코드, 상태 텍스트)
- HTTP/1.1 **404** **Not Found**
- 헤더
- "Content type"
- 없음
- Response 본문 내용이 HTML 인 경우
- Content type: text/html
- Response 본문 내용이 JSON 인 경우
- Content type: application/json
- "Location"
Location: <http://localhost:8080/hello.html>
- Redirect 할 페이지 URL
- "Content type"
- 본문
- HTML
- <!DOCTYPE html> <html> <head><title>By @ResponseBody</title></head> <body>Hello, Spring 정적 웹 페이지!!</body> </html>
- JSON
Response 메세지
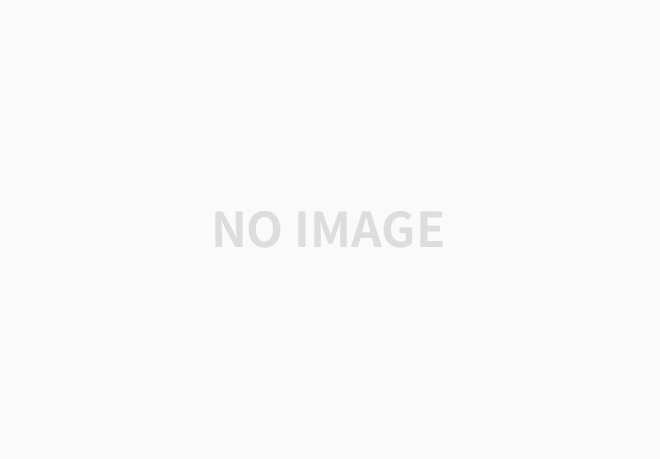
정적 웹페이지
Redirect
@Controller
@RequestMapping("/hello/response")
public class HelloResponseController {
// [Response header]
// Location: http://localhost:8080/hello.html
@GetMapping("/html/redirect")
public String htmlFile() {
return "redirect:/hello.html";
}
}
localhost:8080/hello/response/html/redirect를 입력하면 return 값으로 "redirect:/hello.html"을 보내주었기 때문에 static폴더의 hello.html을 띄워준다.
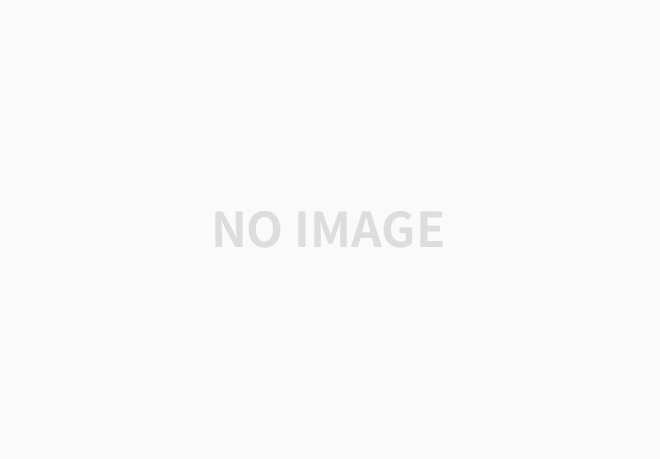
@GetMapping("/html/templates")
public String htmlTemplates() {
return "hello";
}
return 값이 String 타입 -> 템플릿 엔진으로 넘어간다
Template engine 에 View 전달</aside>타임리프 default 설정
- prefix: classpath:/templates/
- suffix: .html
resources**/templates/hello.html**
- @GetMapping("/html/templates") public String htmlTemplates() { return **"hello"**; }
- <aside> 🌐 http://localhost:8080/hello/response/html/templates
@GetMapping("/body/html")
@ResponseBody
public String helloStringHTML() {
return "<!DOCTYPE html>" +
"<html>" +
"<head><meta charset=\"UTF-8\"><title>By @ResponseBody</title></head>" +
"<body> Hello, 정적 웹 페이지!!</body>" +
"</html>";
}
컨트롤러에서 넘겨준 그대로. String 자바의 요청 처럼 + 연산자, 템플릿 엔진으로 넘어가지 않고 바디(본문)에 넣어준다.
동적 웹페이지
@GetMapping("/html/dynamic")
public String helloHtmlFile(Model model) {
visitCount++;
model.addAttribute("visits", visitCount);
// resources/templates/hello-visit.html
return "hello-visit";
}
<div>
(방문자 수: <span th:text="${visits}"></span>)
</div>
JSON 데이터
1. 반환값: String
@GetMapping("/json/string")
@ResponseBody
public String helloStringJson() {
return "{\"name\":\"BTS\",\"age\":28}";
}

2. String외 JAVA 클래스
@GetMapping("/json/class")
@ResponseBody
public Star helloJson() {
return new Star("BTS", 28);
}


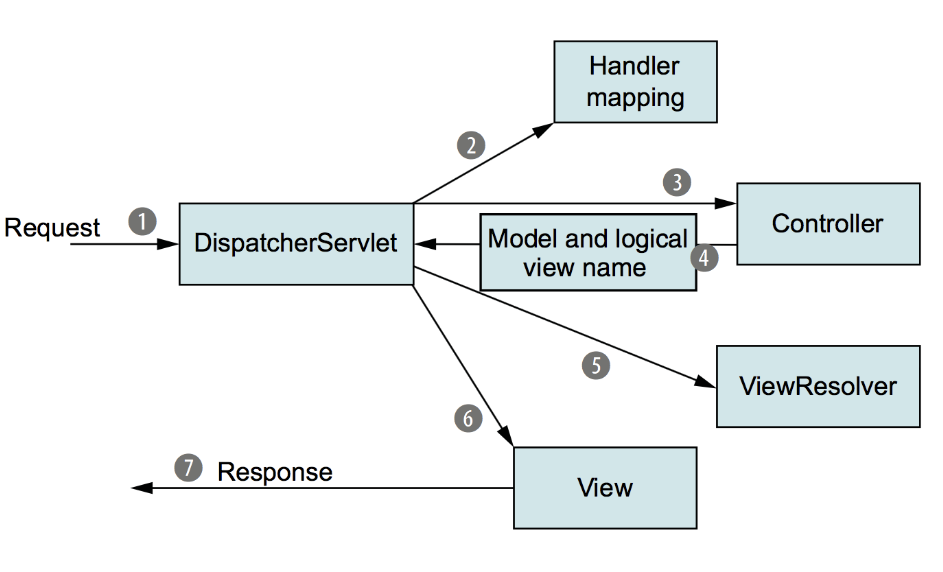
Request
@AllArgsConstructor
@Getter
@Setter
public class Star {
String name;
int age;
}
ModelAttribute를 사용할 때 객체에 @Setter 설정 필수.
@PostMapping("/form/model")
@ResponseBody
public String helloRequestBodyForm(@ModelAttribute Star star) {
return String.format("Hello, @RequestBody.<br> (name = %s, age = %d) ", star.name, star.age);
}
application/json을 받기 위해서는 @RequestBody(생략 불가)를 사용해야 한다
@Controller
@RequestMapping("/test")
public class LoginController {
@GetMapping("/login")
public String getLogin(){
return "redirect:/login-form.html";
}
@PostMapping("/login")
@ResponseBody
public String postLogin(Model model, @RequestParam String id, @RequestParam String password ){
if(id.equals(password)){
model.addAttribute("loginId", id);
}
return "login-result";
}
}
이건 안되고 왜
@Controller
public class LoginController {
@GetMapping("/login")
public String getLogin(){
return "redirect:/login-form.html";
}
@PostMapping("/login")
public String postLogin(Model model, @RequestParam String id, @RequestParam String password ){
if(id.equals(password)){
model.addAttribute("loginId", id);
}
return "login-result";
}
}
RequestMapping을 뺀 이거는 PostMapping이 작동할까...
아 login form에서 action이 "login"으로 되어있기 때문이었다.
<form action="test/login" method="POST">
<div>
아이디: <input name="id" type="text">
</div>
<div>
비밀번호: <input name="password" type="password">
</div>
<button>로그인</button>
</form>
Spring Default 설정에서
static/index.html을 자동 설정
- "절차적 프로그래밍"
- 초기 프로그래밍 방식
- 컴퓨터가 해야할 일들을 쭈~욱 순차적으로 나열해 놓는 코딩 방식
- 예) AllInOneController 클래스의 각 API 처리내용
- "객체지향 프로그래밍"
- 소프트웨어의 규모가 점점 커지면서 필요성이 부각이 됨
- 대부분의 사람들은 한 번에 여러가지 다른 생각을 하는데 취약
- 하나의 사물 (객체) 에 하나의 의미를 부여하는 것처럼 프로그래밍하게 됨
- 예)
- 뭔가 자를 것이 필요하면 '✂️' 를 떠올림 (class Sciccors)
- 종이에 적을 게 필요하면 '✏️' 을 떠올림 (class Pen)
- "하나의 역할" → 객체
- 예)

1. Controller
- 클라이언트의 요청을 받음
- 요청에 대한 처리는 서비스에게 전담
- 클라이언트에게 응답
2. Service
- 사용자의 요구사항을 처리 ('비즈니스 로직') 하는 실세 중에 실세!!!
- 현업에서는 서비스 코드가 계속 비대해짐
- DB 정보가 필요할 때는 Repository 에게 요청
3. Repository
- DB 관리 (연결, 해제, 자원 관리)
- DB CRUD 작업 처리
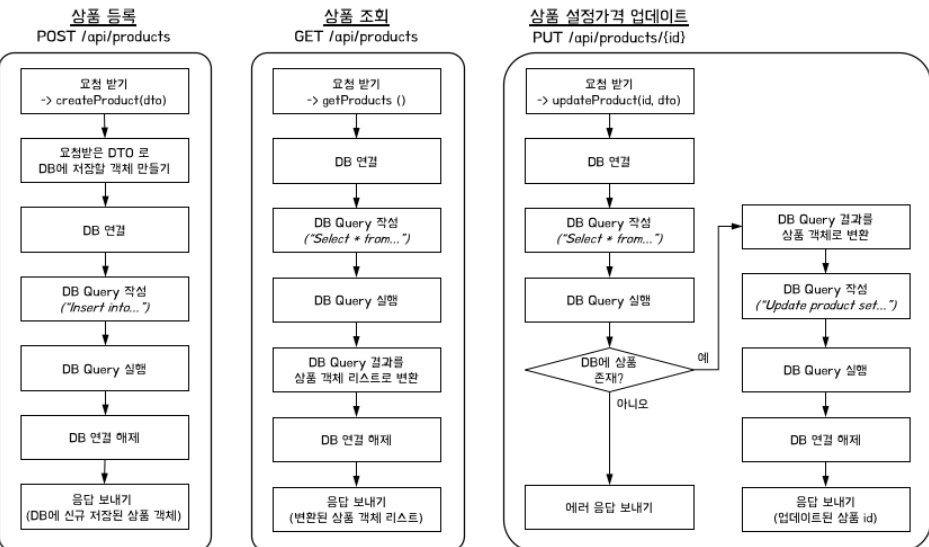
'TIL(Today I Learn)' 카테고리의 다른 글
TIL 220531 (0) | 2022.06.01 |
---|---|
WIL 3주차(Week I Learn) (0) | 2022.05.30 |
TIL 220526 (0) | 2022.05.26 |
TIL 220520 (0) | 2022.05.21 |
TIL 220518 (0) | 2022.05.19 |